How To Build Beautiful Maps With Folium and Leaflet
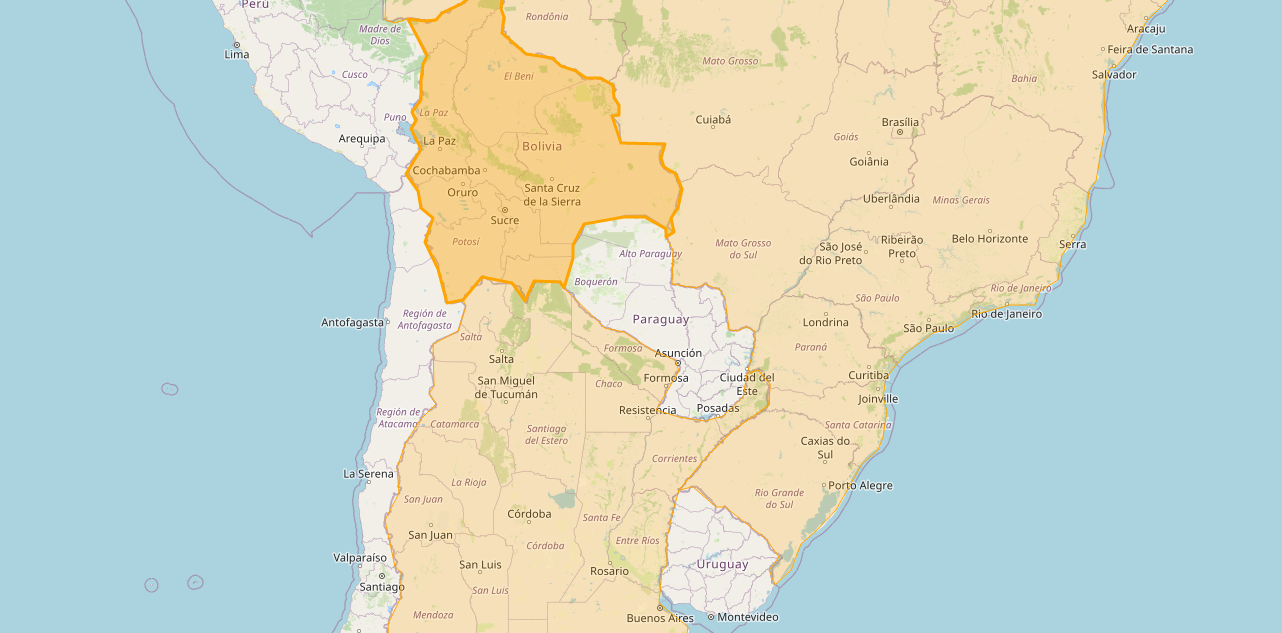
If you like working with data you’re probably very familiar with Python and you know about the power of visualizations to make the data accessible to your readers.
However Python is not the best tool for visualizations. Enter Leaflet, an open-source JavaScript library which creates mobile-friendly maps, has a small footprint, comes with a lot of map layers out of the box and has several more amazing features.
What better way to combine the power of Python and Leaflet? In this short article we’ll create an interactive world map with highlighted countries with the help of Folium and Leaflet.
The Code
Get started by installing Folium with your choice of package-management system, I like to use pip
:
$ pip install folium
Let’s continue by getting some data, Folium provides some sample data, e.g. a list of countries with polygon coordinates for their borders, you can grab it like this:
$ wget https://raw.githubusercontent.com/python-visualization/folium/master/examples/data/world-countries.json
Next read this data into a dictionary so that we can further process it, we’re going to use Python 3.8.0 throughout this article:
import json
with open('world-countries.json', 'r') as f:
world_countries = json.loads(f.read())
print(len(world_countries['features']))
In my example I want to highlight countries I’ve visited (I use this on a separate page), so from the 177 features we got in our source only keep the ones we’re interested in:
# Remove countries we're not interested in
visited = ['Argentina', 'Brazil', 'Bolivia', 'Germany']
new_features = []
for feature in world_countries['features']:
if feature['properties']['name'] in visited:
new_features.append(feature)
new_world_countries = world_countries
new_world_countries['features'] = new_features
print(len(new_features))
Great! Now all that’s left is to add our data to a Folium map and get the resulting Leaflet code:
import folium
m = folium.Map(location=[51, 0], zoom_start=2.5)
folium.Choropleth(
geo_data=new_world_countries,
highlight=True,
fill_color='orange',
fill_opacity=0.2,
line_color='orange'
).add_to(m)
folium.LayerControl().add_to(m)
This first instantiates a new Folium map with folium.Map
, we pass it a starting location and zoom level. Next we form a choropleth with folium.Choropleth
, define what data to use, whether to highlight, and the highlight options, finally we add this to our Folium map.
All that’s left is to render the Folium map:
html_string = m.get_root().render()
print(html_string)
We end up with HTML which we can embed anywhere we like, e.g. I added it below.
Conclusion
Those few lines of code bridge the gap between your favorite data wrangling programming language and beautiful, fast, and highly interactive maps with JavaScript.
Now that you have the tool set to connect your Python datasets with powerful maps go and explore what Folium has to offer and let me know of any amazing maps you build.